Intro to Labview :
LabVIEW (Laboratory Virtual Instrument Engineering Workbench) is a graphical
programming environment which has become prevalent throughout research
labs, academia and industry.
It is a powerful and versatile analysis and instrumentation software
system for measurement and automation. Its graphical programming language
called G programming is performed using a graphical block diagram that
compiles into machine code and eliminates a lot of the syntactical details.
Whenever you will open labview, Goto File>New Vi.
You will see two windows open.
The one with lines is Front Panel. The one with white background
is block panel.
What is Front Panel?
Front Panel is basically where you will make the GUI of your program.
Some basic things to add to your front panel can be like LED , Graph ,
Indicators etc..
What is Block Panel?
Block panel is where you will do all the coding. Well not some coding
like C or Java or C#. But you will do Graphical Programming in which you
will have to attach to different wires to create a logic.
What are Controls, Indicators, Constants?
Controls-These are basically variables whose value we can change at the
time when our program is running. These mostly act as input to a function.
Indicators- These are used to show the output of some function whether
the result to be shown is Boolean or numeric or string.
Constants- These are constants like in normal programming whose value
we cannot change while our program is running. These are also used as Input
to a program.
Now let us see the basic use of these by an example:
To add two numbers:
1. On block panel ,Right click to open function palette. Then
click on numeric. Drag the Add function
to block panel.
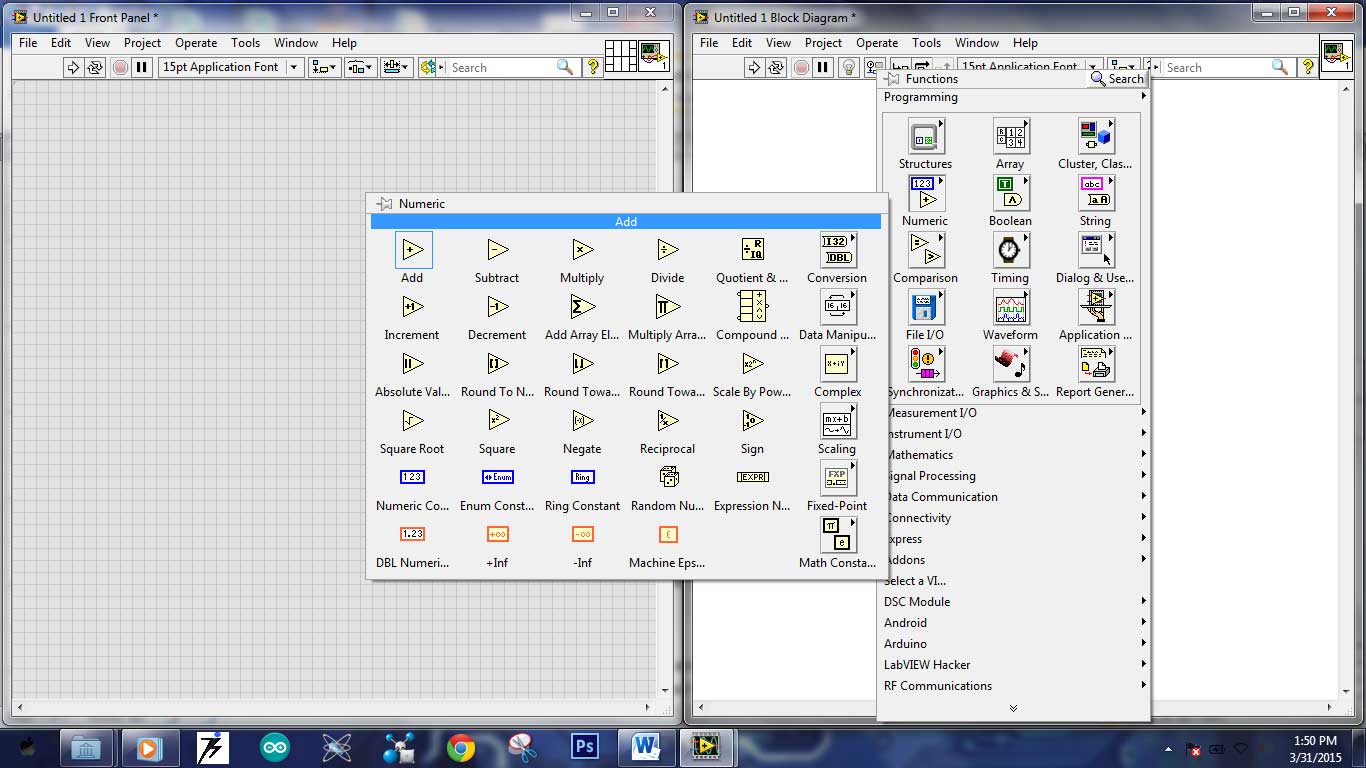
2. Now you have to define two variables to add. Right Click on Front panel,
Goto Numeric and Drag the First numeric control to front panel. Drop another
one to define second variable.
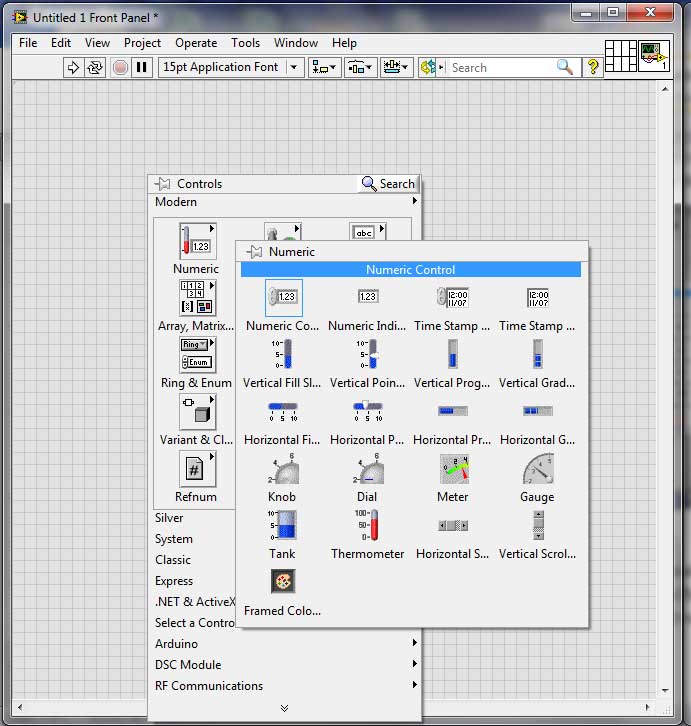
3. You will notice that there are two blocks on block panel now. Now bring your mouse close to the arrow shaped part of block, its symbol will change to

4. When the symbol appears, click and attach it to the addition block input part. Do the same with second variable.
5. Now to display the result of two numbers. On Front panel, Drag a Numeric indicator. Attach the output of add function to arrow part of indicator block that appeared at block panel.
6. Lastly, just press running continously button.
Now the vi is running continously. Change the values of controls on Front
panel and you will notice the change in output value.
Loops:
Labview has same facility as any other programming language to use for
loop,while loop etc..
In the same way, just drag the while loop to your block panel which
available under structure section in function palette.
Comparison operators:
There are all kind of operators like greater than, less than, equal to
etc. These operators output in true or false. So to see the result just
drag a Boolean led in front panel and attach the output of operator to
the block formed by led in block panel.
Logical operators:
As we have an option of performing operations like logical AND or OR operation
in C or Java, in same way we have these logical operators. In this,we have
to give Boolean inputs which will further give output in Boolean. These
operators are available under Boolean section of function palette in block
panel.
Shift Register:
The main advantage of adding a shift register in labview is to able to
pass the values in feedback way. It can be added to while loop by right
clicking on it and then click on Add shift register. It will look like
this.
A short example of use of shift register:
Let us we want to blink an LED:
1. Create a new vi. Drag a while loop and add shift register as stated
above.
Create Stop button for while loop by right clicking
on red button and selecting
create CONTROL
2. Lets drag a Boolean indicator say Round LED by Right Clicking on Front
Panel, under Boolean Options.
3. A counterpart would have formed in block panel. Right click on it and
select “Change to Control”. Attach its output to shift
register.
4. After attaching Boolean, the color of shift register will change to
green like this:
5. Now we need to drag a NOT GATE from block panel side under Boolean.
Attach the inside of Shift Register to NOT GATE.
Also add a delay of 1sec using Wait ms block.
6. Take another LED and lets change its name to BLINKING. Attach the output
of NOT GATE to this LED and to the other part of
shift register like this:
7. Now Run the Vi and you will see the led blinking.
Explanation:
The Boolean led is to initialize the shift register with logic FALSE.
This value is passed to Not Gate and it inverts this to logic TRUE.
This inverted logic TRUE is passed to BLINKING Led and to the other
end of shift register.
The BLINKING Led Turns on and side by side that logic gets shifted to
the other shift register part which is at front.
Now Shift Register holds TRUE logic and hence this time Led turns
OFF after a delay of 1sec or 1000msec. This programs runs infinetly unless
you press Stop button.
Local Variable:
In same way as C language, we can create local variables which can be
only accessed in one program(VI). We cannot use local variables in another
programs. Local variables can implemented in controls available in our
current program. Local variables are a kind of storage where the value
of control is stored.
1. Let us create a control and change its name to say ‘a’.
2. Right Click on block panel and then under Structures, drag the
local variable.
3. When you will hover over local variable, mouse symbol will change to
hand, then click on it and select ‘a’ which was
our control. The symbol of our local gets
changed now. Now the local variable will store
the current/whatever maybe the
value of ‘a’.
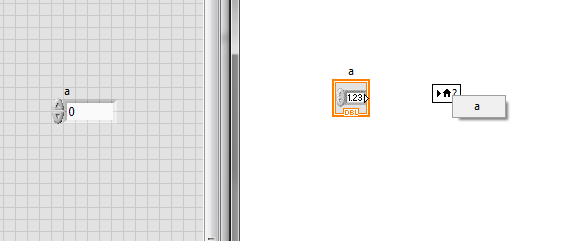
Global Variable:
In same way as local ,we can create global variables which can be accessed
in any program. Rather than having local scope to only in one program,
we can use this in simultaneously any number of running VI’s.
Let us create a global variable in same way as local.
1. Just drag the global variable on to block panel from under Structures.
Double click on global variable and it will
pop-up/create new front panel.
2. Now create 2 controls and name them ‘a’ and ‘b’. Put some values in
it say 5 and 4 resp. Save the vi as global.vi
and close its front panel.
3. After saving the vi, just hover over previously global variable and
when then hand comes just select any of ‘a’
or ‘b’ control in that variable.
4. Just copy the global variable and select ‘b’ now.
5. Right click on global variables and click on ‘change to read’ because
we want to read these variables
6. Create the two indicators in front panel and attach these to the output
of global variables so as to their value.
Select Function:
Returns the value wired to the t input or f input, depending on the value
of s.
If s is TRUE, this function returns the value wired to t . If s is
FALSE, this function returns the value wired to f. It’s is like conditional
operator in C language. Or you can say like if Condition.
As there is no direct way of using if condition,we can use this function.
Example:
Let’s say we want to display the square root of a number. But if the number
is –ve then it should display some constant value:
1. Drag the Select Function by Right Clicking on Block Panel,under comparison,choose
Select funciton.
2. Now we need to check whether a number is +ve or –ve. For that drag
less than(<) operator from under comparisons. To one termianl of it,
wire a Numeric control by creating one on Front Panel. To other,wire a
constant 0.
3. Now the condition is set, we need to provide false and true results.
For TRUE, drag a square root from numeric funcitons. Wire it with numeric
control and it’s output to true terminal of SELECT function
4. For FALSE result, drag a constant from under NUMERIC funcitons. PUT
-9999 value in it. Wire it to the false terminal.
5. Create a indicator by hovering your mouse over output of SELECT
Function. Then right click on it and select create indicator. Run the vi
and change the value of control from Front Panel and see the Square Root
as result. When the value drops less than 0, it outputs the false result
which is -9999.
Formula Node:
This is basically like your C language block in which you can write your
C Code but not too complex one. This is used only in minor ways.
You can create as many inputs variables and output variable by right clicking
on formulae node.
Let us understand this by making a simple if else program and output the
larger number.
1. Create a Formula Node From under Structures in Block Panel
2. Create two Numeric Controls in Front Panel.
3. Right Click on the left side of Formula Node and Select “ADD INPUT”.
Do it Again.
4. Now From the right side,”ADD OUTPUT”.
5. Now Attach the both Controls to inputs of Formula Node and to output,
Attach an indicator.
6. Change the names of Controls if you want. Now for the important part,
Double click inside the one of INPUTS. Now you will see Cursor blinking
inside the node. Give a variable name like ‘a’ for the 1st control.
7. Similarly give ‘b’ for the second. And ‘y’ for the output.
8. Now comes the coding part. It’s not like it is too hard. Just some if else part.
9. Run the vi and change a to 2. As you can see in the figure, y has value 2 which was in a. So it means the 1st conditon is satisfied. Try changing b value to see different output.
Case Structure:
Contains one or more subdiagrams, or cases, exactly one of which executes
when the structure executes. The value wired to the selector terminal(
which looks liks (‘?’) determines which case to execute. It is like a switch
case of C language.
In normal case, case structure has two conditions. TRUE or FALSE.
If we wire the Boolean control to selector terminal, if Boolean has true
logic, then case under TRUE subdiagram will execute and vice-versa.
You can browse through cases by clicking the arrow next to where True
is written.
For example, Lets execute a addition when boolean or LED is on and Subtraction
when LED is off.
1.Drag a Case Structure from under Structures in Block Panel.
2. By default, True case will appear. Now Create two numeric controls
and one indicator and name as a,b,y resp. in Front Panel.
3. For addition, drag addition function and wire as shown below:
4. Change the case by clicking the arrow at middle of case structure.
Now you will see false written on it.
5. Do the same but drag subtract function this time as shown below:
6. Attach the Boolean control by creating one to the selector terminal
and Run the vi.
7. When the LED is on, addition case will run.
Event Structure:
Just like Case Structure, Event Structure also contains cases but different
is that you can assign a time for event to run by creating a constant of
defined milliseconds by wiring it with above Sand Time symbol.
Let’s understand it by an example same as above but now having two Boolean
controls for each event.
1. Create two Boolean controls. Drag an event structure. Now right click
on the arrow near Timeout and select choose “ADD EVENT CASE”. You will
see window like this:
2. Select Boolean as in above figure and click ok. Now event is added.
Add another event but select Boolean 2 this time.
3. From above select “Boolean” event from arrows. As above example,
create two controls and an indicator. Drag that Boolean control inside
that Event. Do the addition here as shown below:
4. Change the event to Boolean 2 and do subtraction this time. Drag Boolean
2 control inside this event.
5. Run the vi and Turn LED on or off to see addition or subtraction
resp.
Note: You will notice that at 1st time, you have to switch on and off an led to see that event occur. But if you now click on 2nd LED, then that event happens immediately Because previously some event has already happened.
Waveform Chart:
The waveform chart is a special type of numeric indicator that displays
one or more plots of data typically acquired at a constant rate.
You can drag a chart from Front Panel. Go to Graph->Waveform Chart.
There are three types in which you can update your chart.
You can see the update option when you will Right Click on the chart
in Front Panel. Go to Advanced->Update Mode->Strip/Scope/Sweep.
1. Strip chart: Shows running data continuously scrolling from left to
right across the chart with old data on the left and new data on the right.
A strip chart is similar to a paper tape strip chart recorder . The strip
chart is the default update mode.
2. Scope Chart: Shows one item of data, such as a pulse or wave, scrolling
partway across the chart from left to right. For each new value, the chart
plots the value to the right of the last value.
When the plot reaches the right border of the plotting area, LabVIEW
erases the plot and begins plotting again from the left border. The retracing
display of a scope chart is similar to an oscilloscope.
3. Sweep Chart: Works similarly to a scope chart except it shows the old
data on the right and the new data on the left separated by a vertical
line.
LabVIEW does not erase the plot in a sweep chart when the plot reaches
the right border of the plotting area. A sweep chart is similar to an Electrocardiogram
(EKG) display.
If you want to clear the chart, Right Click on Chart and Go to Data Operations->Clear
Chart.
If you want to change the range as in above is between 10 and 20. Double
click on the 10 above and enter the initial range and vice-versa.
Let us see an example:
1. Drag a chart from Front Panel. On the block Panel side, drag a
random number from Under Numeric in block Panel.
2. Attach the Output of random number to chart in Block Panel.
3. Add a while loop with 1sec delay .
4. By default, the chart will be in Strip Mode, random number value between
0 and 1, x-axis and y-axis values will be dynamic(means they change as
the chart moves.
5. If you want to make the x-axis static, right click on chart in Front Panel. Go to Properties->Scales(tab)->Tick mark on Autoscale. Now only the Final value of axis will increase.
Waveform Graph:
The waveform graph displays one or more plots of evenly sampled measurements.
The waveform graph plots only single-valued functions, as in y = f(x),
with points evenly distributed along the x-axis, such as acquired time-varying
waveforms.
It is same like waveform chart but we will usually use it to display
waves like sine, cosine etc.
It does not have any update options. It is available under same option
in Front Panel like Waveform Chart. .
Let us understand it by an example: We will simulate sine wave on the
graph.
1. In Block Panel, Right click and go to Signal Processing->Waveform
generation->Simulate signal(drag it).
2. You will see a window will open. Just click on OK for now. By
default the sine wave is chosen.
3. Attach the output of this with graph in Block Panel. Add a while loop
and a delay of 1sec. In one sec, 100 samples are there by default.
Multiple Signals in waveform Chart:
1. Go to Search box above and type Merge signals. Drag it to the Block
Panel.
2. Now add two random number functions and chart. Attach them to Merge
signals and merge signal output to the Chart .
3. Add a while loop and a 1sec delay.
4. With Graph, Create two Simulate signals. In one Change the option of Phase from 0 to 90 . This will create a cosine wave.
Write to file:
Sometimes you need to save the data to an external file say TDM,CSV or
Binary.
LabVIEW can use or create the following file formats: Binary, ASCII, LVM,
and TDM.
• Binary—Binary files are the underlying file format of all other file
formats.
• ASCII—An ASCII file is a specific type of binary file that is a standard
used by most programs. It consists of a series of ASCII codes. ASCII files
are also called text files.
• LVM—The LabVIEW measurement data file (.lvm) is a tab-delimited text
file you can open with a spreadsheet application or a text-editing application.
The .lvm file includes information about the data, such as the date and
time the data was generated. This file format is a specific type of ASCII
file created for LabVIEW.
• TDM—This file format is a specific type of binary file created for National
Instruments products. It actually consists of two separate files: an XML
section contains the data attributes and a binary file for the waveform.
There are two ways doing this.
1. By using Write to Measurement file
2. By using Write to Spreadsheet file.
We will see both options here. Let us understand it by an example:
By Using Write to Measurement file:
1. Do the random number experiment as we did above. Now we want to save
the values in Excel say.
2. Right Click on Block Panel and Go to File I/O->Drag Write to Measurement
File. 3. A Window will Pop-Up. Choose the options as shown below:
4. In the Filename, select the location where you want to save the file
and give it a name. The file will be created automatically. Click on Ok.
5. Run the vi for like 10 sec and then open that file to see the
values.
By using Write to Spreadsheet file:
1. Again do the random number experiment as above. Add Write to Spreadsheet
File from File I/O in Block Panel.
2. When you will see the explanation of this block (by clicking on
the question mark on top right-side and then clicking on Write to Spreadsheet
File block). You will notice that it accepts only 1D or 2D data. So we
cannot directly connect random number to this block.
3. To convert into 1D data, we have to store it in 1D array. For that,
Right Click in Block Panel, Go to Array->Build Array. This block is
not as flexible as Write to Measurement file. We have to create some options
to make it work.
4. We have to set file path, 1D data, append, delimiter pin of Write
to Spreadsheet file. See these in figure below.
5.a File Path: Create a New Text Document in Desktop. Name it as data and change its extension to ‘.csv’ . From the Front panel select the path as shown below:
5.b 1D data: Attach it as shown below:
5.c Append: Hover over append pin of this block. Right Click on
it and select create constant. Change it to true by clicking on it.
5.d Delimiter: In some way, create constant by hovering and selecting
it. Double click inside that constant and write comma(,) there.
6. Now that everything is ready, run the vi for 10 sec and see the results:
Hardware with LabVIEW:
Softwares needed:
1. LabVIEW 2013
2. VIPM- LIFA and Makerhub LINX
3. VISA
4. Arduino IDE
We will download
VIPM( Virtual Instruments Package Manager) so that we can various add-ons
that are Labview. The ones that we need are LIFA and LINX.
Next is a Software/Driver
VISA which helps in interfacing Hardware like Arduino(in this case) with
Labview.
From Now on, we will be using Arduino as the only hardware interface with
LabVIEW.
Once you have downloaded and installed VIPM 2014, it is time to configure
it with LabVIEW 2013 so that we can download LIFA and LINX.
Configuring VIPM with LabVIEW:
1. Open VIPM after installing it. Make sure that your internet connection
is on. It might take some time for it to start for the 1st time as it has
to download all the libraries.
2. Once it is done downloading, it will look like this.
3. Now we to set it up with LabVIEW. For that, open LabVIEW and Go to Tools->Options->VI Server.
4. Fill the Options as shown below by adding localhost,127.0.0.1,* to Machine Access List and * to Exported Vis.
5. After Saving the Options, open VIPM and Go To Tools->Options->LabVIEW (tab). As in Figure below, you can see that Connection is verified. Tick mark the LabVIEW version you have and click on verify.
6. If everything goes right, connection will be verified. Make sure that
NO OTHER software like WAMP SERVER or service is using localhost that time
or it will fail. After verification, you will be able to download the add-ons.
7. Make sure your internet connection is fast. In the search bar
of VIPM, type Labview interface for Arduino. Double click on it. Follow
the instructions and install it. In same way, install Makerhub LINX.
8. Open your LabVIEW. You will see options like these now:
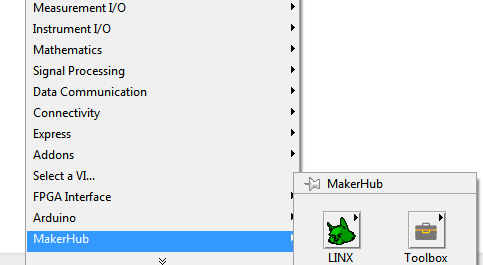
Configure VISA with LabVIEW:
Next step is to install VISA software which is
here
It is just plain and simple. Download and install it.
It is required so that whenever we attach a hardware, it must show up
in the labview as a com port which you can check in Device Manager.
Installing Arduino IDE:
Arduino IDE . Most of the time i would like to use Arduino 1.5 in Window
7 because it never gave me an error.
If you want to learn about arduino, please check the Arduino tutorial
section
here.
Dumping LIFA BASE in ARDUINO:
For Arudino to work with labview, we have to dump a program in arduino
using Arduino IDE. After installing LIFA from VIPM, it gets installed here-
1. (C:\Program Files (x86)\National Instruments\LabVIEW 2013\vi.lib\LabVIEW
Interface for Arduino\Firmware).
2. Open the LIFA BASE folder. Double click on Lifa_Base.ino and it should
open Arduino IDE.
3. Now click on right-arrow button and now the program will dump in Arduino.
LED Glow using LIFA.
It is really a simple task of glowing an LED in arduino from LabVIEW.
We will use LIFA in this case but it is no more different than using
LINX.
LIFA is deprecated and is no more updated but i still prefer it because
of its more features.
Once you have downloaded LIFA BASE in Arduino, proceed as said:
NOW let us start by Right Clicking in Block Panel.
1. Go to Arduino->Init ( drag it). Then Go to Arudino->Low Level->Set
Digital Pin Mode( drag it). Again Go to Arudino->Low Level->Digital
Write( drag it). Now again drag the Close block in Arduino. Join them as
shown below.
2. Now right click on Visa Resource pin of Init block and select Create
Control. If you have some other Arduino, change the Board type from init
block by Creating Constant on the pin.
3. Choose the pin on which you want to blink an LED, let us take
8th pin. Then Create a constant on Digital I/O pin of 2nd Block. Combine
it with same pin of 3rd block. Put 8 in Constant.
4. In the Pin Mode pin, create a constant and choose output by clicking
over it. In the value pin of 3rd block, we will add Boolean control to
switch on/off led. Drag Boolean to (0,1) function from Boolean in Function
Palette to convert TRUE/FALSE to 0,1 which is accepted by Value Pin and
add a while loop.
5. Drag Unbundle by Name from Under Cluster and a OR gate so that
whether there is an error or user clicks stop button, the vi will stop.
6. Drag a simple error from under Dialogs & User Interface.
7. When you have connected to PC, a COM PORT will be assigned to it. Check this by searching DEVICE MANAGER in windows. In device manager, see under PORTS. 8. Now you know COM PORT, Click on the arrow in Visa Resource control and choose that COM PORT. Run the vi. If there is no error, you will be able to control a led from Boolean control.
Blinking an LED using LIFA:
By keeping the same steps as above and taking into account the use
of shift register, this can be easily done:
1. Add a Shift Register in while loop and a Not Gate.
2. Boolean will act as initial control to put value in Shift Register.
Suppose initially Boolean control is OFF or has FALSE state. Now this state
will converted to 0 and passed to the DIGITAL WRITE block.
3. In the same time, that state will be inverted by NOT GATE and
passed further to other end of shift register.
4. This state i.e TRUE will be passed as initial state and this time
it gets converted to 1 and LED at 8th pin will be ON.
5. You can add delay of 1sec to blink the LED slowly as shown below:
6. Run the vi and see the result on your Arudino Uno.
Controlling LED Intensity using LIFA:
By keeping most of the steps as above, we need to add/replace Digital
Write block with PWM WRITE block from Arduino->Low Level in Functions
Palette.
PWM pins in Arduino UNO are as 3,5,6,9,10,11. So you need to connect
led to one of these pins.
1. To Control intensity, we need to create a CONTROL in Front Panel whose
range varies from 0 to 255. Therefore, drag a Knob from under Numeric in
Front Panel.
2. By default, know has range from 0 to 10. Change it to 0 to 255 by double
clicking on the final range value and entering 255. But this Know gives
values as floating number. We need to convert it to integer because pwm
occurs on proper integer values.
3. Therefore, Right Click on Knob in Front Panel and Go to Representation->I64(select
it). Now knob will give integer values.
4. Attach the Knob with Duty Cycle pin of PWM WRITE block. Change
the digital pin to 9 (or any of above) and attach it to 2nd pin of that
block as shown below:
5. Connect the led with 9th pin of Arduino. Choose the COM PORT. Run the vi and change the knob position to see the change in intensity.
Analog read, waveform chart and data storing using LIFA:
If you have want to read the analog value of ldr or any sensor that
is connected to Analog Pin of Arduino, then we will use Analog read pin
block this time.
Arduino has 10bit ADC and has logic level from 0 to 5V meaning that
lowest value of LDR is 0 and maximum is 1023 or 5V.
Analog read pin block reads this value b/w 0 to 5V in labview for
the varying values of LDR. Hence the Maximum Value of Waveform Chart will
be 5V.
If say you have a Temperature Sensor attached to Arduino then you
have to calibrate that value in labview to get the temperature.
Now the steps that involved are:
1. By following the most of steps as above, we need to remove the
Set digital pin mode and Digital Write block. In Arduino, analog pins are
input by DEFAULT.
2. Connect the LDR or Variable Resistor or any other sensor to Analog
Pin of Arduino say A0 pin.
3. Connect the Analog read pin block and waveform chart as shown
below:
4. Choose the COM PORT. Run the vi and value will be read and displayed
in waveform chart with 1sec delay.
5. Now let us see how we can store this data into a Spreadsheet file.
We know that there are two ways to store data. Using Write to Measurement
or Write to Spreadsheet.
6. I will use Write to Spreadsheet like we did before here(Give link here).
Drag two Write to Spreadsheet block from under File I/O. One will be used
to give the sensor name and other for the values.
7. Go to Front Panel->Array, Matrix & Cluster->Array(drag
it). Now drag a String Control from under String & Path.
8. After doing that, you need to drag this string Control inside
Array and enter LDR. It will look like this:
9. After Configuring all the pins like here, it will look like this:
10. Create data.csv file at Desktop and give it’s location. Drag Build
Array in Block Panel and connect it with value output of Analog pin and
1D input of 2nd Spreadsheet block.
11. After Combining it with our programme, it will look like this as a
whole.
12. Choose the COM PORT. Run the vi and change the value of sensor to see the change in value . Waveform chart and csv file is shown as below:
Led Blinking using LINX:
The steps are same as of LIFA. Only the blocks are needed to be changed.
Steps are:
1. Upload the LINX Frimware in Arduino by going to TOOLS->Makerhub->LINX->LINX
Firmware Wizard. Choose your device, connection type and COM PORT.
2. Once the Firmware gets uploaded, drag the initialize block from under
MakerHub->LINX in Block Panel.
3. Drag Write Block from MakerHub-> LINX->Peripherals->Digital->Write.
4. Now drag the Close block. Connect them. It will look like this.
5. Configure the pins of each block as shown below:
6. Drag the while loop, a delay block and a local variable as shown below:
7. We will not use shift register this time to blink led, instead we will use Local Variable. Now the local variable is empty. We need to provide value to it. So create a Boolean Control and then change local variable to Boolean by clicking on it. Drag a NOT Gate and connect them. Also add a error control as shown:
8. Choose the COM PORT. Run the Vi and you will see the led blinking now. In vi, Led is off for the 1st time and it passes false logic to value pin of write block. At the same time value gets inverted by not gate is passed to local variable. But this local variable and Boolean control share same storage. So now, the false logic will be overwritten. This time the led will get on.